Subscriber - Registry

This module provides some base classes, to implement a registry/subscriber system, allowing to be aware of the availability (based on spawn and custom criteria) of remote objects, and making the order in which objects spawn transparent.
This systems ensures that:
- all clients are notified when a subscriber registers to one or several centralized registries
- a subscriber registration/unregistration is dependent on a
IsAvailable
property (registration requires to be available, and by default unavailbility triggers unregistration). While not networked, this property value should in general be based on networked values so that the registration triggers at the same time on all clients
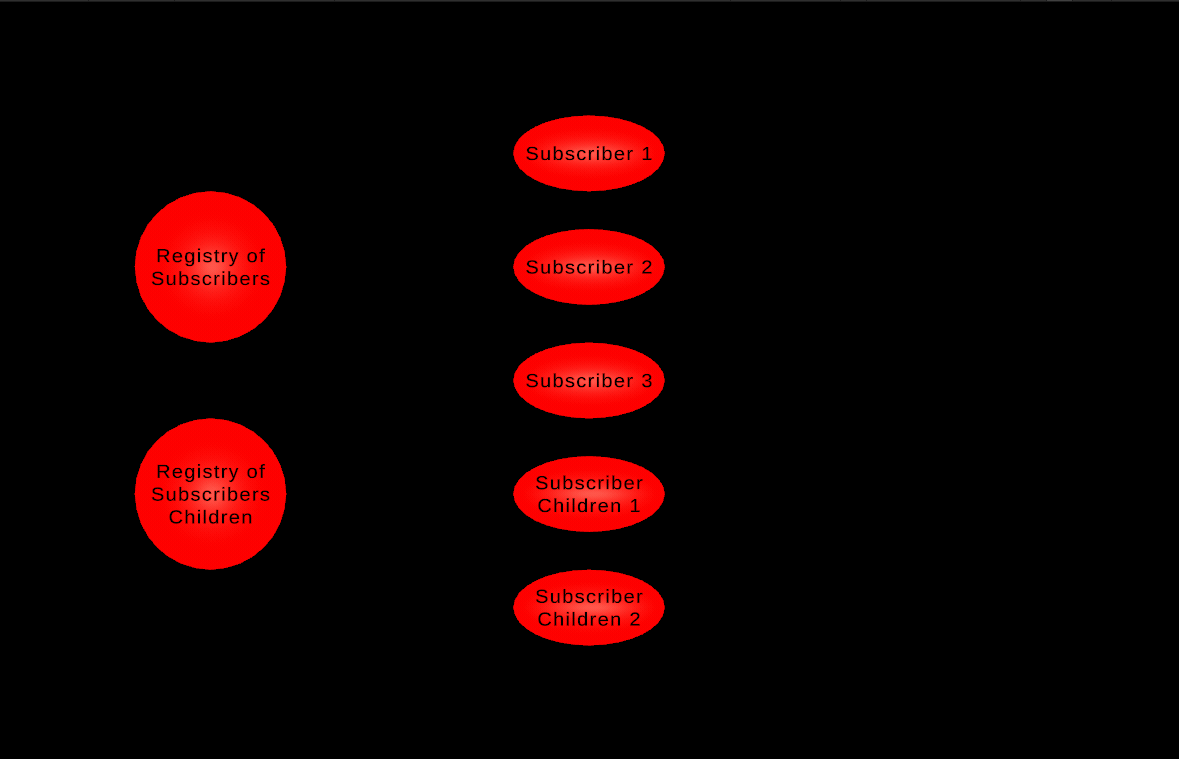
Core logic
The common usage is that the scene contains a Registry as a scene networked object, while players spawn a subscriber when they connect.
The order in which the Registry or the various Subscriber spawn for a late joiner is not always the same. The Subscriber/Registry classes make sure that it does not matter, by ensuring that a subscriber registers to a registry only when both of them are available.
It includes both:
- being spawned,
- but also additional use case specific criteria if needed.
The goal of the add-on is to make sure that the order of 1 to 4 step does not matter:
- The registry spawns
- The subscriber spawns
- The registry becomes available
- The subscriber becomes available
- The subscriber registers to the registry
- Registry and subscriber callbacks are triggered, and potential other listeners are warned
Base classes
Subscriber<T>
and Registry<T>
are generic classes to allow several subclasses to coexists in a same app.
The T
type has to be the Subscriber<T>
subclass itself, for instance class SampleSubscriber : Subscriber<SampleSubscriber> {}
, for the proper typing to apply in method and properties.
Subscriber
A Subscriber<T>
subclass will register to a Register<T>
subclass when both of them are available (IsAvailable
= true). Note that IsAvailable
source criteria needs to be synched to be sure every clients receive the callbacks at the same time.
All clients can receive notification of registration by overriding OnRegisterOnRegistry(IRegistry<T> registry)
/ OnUnregisterOnRegistry(IRegistry<T> registry)
or in another component by implementing RegistryListener<T>
and using IRegisterListeners(RegistryListener<T> listener)
Typical usage for sub-classes:
C#
// When CustomAvailabilityCriteriaMatched will be set to true, the SampleSubscriber registration will trigger
class SampleSubscriber : Subscriber<SampleSubscriber> {
// Custom availability criteria
[Networked]
bool CustomAvailabilityCriteriaMatched { get; set; } = false;
// Availability override. The data to compute it needs to be synched so that the availability triggers everywhere (and hence the registration)
public override bool IsAvailable => base.IsAvailable && CustomAvailabilityCriteriaMatched;
}
Registry
A Registry<T>
will receive T
(Subscriber
subclass) registrations, when both of them are available (IsAvailable
= true).
All clients can receive notifications of registration, either by overriding OnSubscriberRegistration
/OnSubscriberUnregistration
, or in another component by implementing IRegistryListener<T>
and using RegisterListeners(RegistryListener<T> listener)
Typical usage for sub-classes:
C#
class SampleRegistry : Registry<SampleSubscriber> {}
Creating subclasses
A Subscriber<T>
subclass will register to any Registry<T>
(with the same T
type).
If you need to be more restrictive, it is possible to restrict subscriber type by overriding the System.Type RegistryType()
method.
In addition to the OnRegisterOnRegistry(IRegistry<T> registry)
and OnUnregisterOnRegistry(IRegistry<T> registry)
methods that can be overridden to have callbacks on registration, it is possible to override oid OnAvailableRegistryFound(Registry<T> registry)
to be warned when a registry is available (but when the subscriber might not yet be).
Demo
A demo scene can be found in Assets\Photon\FusionAddons\SubscriberRegistry\Demo\ folder.
The scene contains several registers and subscribers. They are activated (the availability variable or the game object) at different timings to show that the registration process is running smoothly despite this.
Dependencies
- XRShared addon
Download
This addon latest version is included into the Industries addon project
Supported topologies
- shared mode
Changelog
- Version 2.0.0: First release