Further Steps
This portion of the documentation will go over a few aspects of Fusion Animal Coop in more detail to help facilitate further education regarding this sample.
Equipment
Players use equipment to interact with the world, like using an axe to chop down trees. This also includes the empty-handed equipment, which has contextual behaviour such as picking up items, shaking trees and speaking to NPCs.
The base Equipment class defines a Use
method which carries out all of the logic for using equipment. With the exception of ShovelEquipment
which has unique behavior, equipment need only implement the InteractionType
and RequiresInteractable
abstract properties to be usable.
C#
[Networked, Capacity(8)] public NetworkLinkedList<Equipment> Equipments { get; }
[Networked, OnChangedRender(nameof(EquipmentIndexChanged))] public byte EquipmentIndex { get; set; }
public Equipment ActiveEquipment => Equipments.Get(EquipmentIndex);
Code snippet from Character.cs regarding equipment.
On the Character, there is a NetworkLinkedList<Equipment>
which is populated in the inspector. The equipment currently in use is dictated by an index into the list, and is resolved via the Get
method. Both properties are Networked so that peers can accurately see what the Character has equipped.
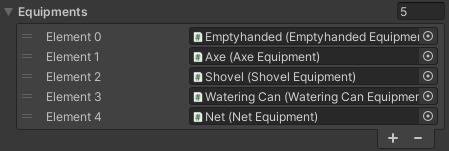
Equipment Interactions
Each equipment defines a type of interaction which they pair with, the InteractionType
mentioned prior.
The InteractionBase
abstract class provides a simple but effective means of executing actions, via exposed events in the inspector. The interaction implementations in this sample are intentionally empty; while they could implement bespoke logic by overriding the Interact
method of the base class, there was no need to do so. The usefulness of the interaction scripts comes from the association between equipment and interaction, and Unity's GetComponent methods.
Both the equipment and the interaction declare an AnimationResponse; the interaction's will be prioritized unless left null, in which case the equipment's will be used as a fallback.
The AnimationResponse contains a string corresponding to the name of a state in the animator, the duration of the animation, and a delay after which the associated action will be performed. Time values are specified in seconds.
Items
All objects which can exist within an inventory have a data representation in the form of an InventoryItem
. This describes both cosmetic and functional aspects of the item, such as the name, icon, and monetary values. Also part of this data is a reference to the item's physical representation, and any interactions that can be performed with it.
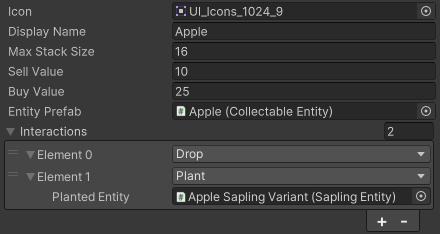
Item Interactions
Items can have any combination of various interactions, which may be performed from the inventory screen when an item is selected. Scripting provides the behavior, and data provides the binding between item and interaction. Any class implementing the IItemInteraction
interface will be automatically made available for addition to items' interaction lists.
C#
public interface IItemInteraction
{
public string ActionName { get; }
public bool CanPerformInteraction(Inventory inventory, InventoryItem item);
public void PerformInteraction(Inventory inventory, InventoryItem item);
}
The signature of the IItemInteraction
interface.
Inventory
The inventory keeps track of items using a NetworkLinkedList. The list contains "slots" which store an ID and a count. Slots are added and removed as needed, and will have their count modified as needed and respecting the maximum stack size as defined by the InventoryItem
data object. Item IDs are resolved through a static-accessible object which provides a two-way dictionary, allowing the querying of an ID given a specific item, and vice versa.
Customization
This sample includes character customization in the form of color and model swaps, facilitated by a custom implementation of an INetworkStruct
. This allows for a single Networked property to control all customization options, minimizing network throughput and code footprint.
C#
public struct VisualData : INetworkStruct
{
public byte skinColor;
public byte topColor;
public byte bottomColor;
public byte hairColor;
public byte hairStyle;
public byte hat;
public byte accessory;
}
The byte datatype is used to minimize data that needs to be sent over the network.
Back to top