Events
Overview
Quantum Animator provides support for Instant
events and Time-Window
events.
Creating a New Instant Event
Events assets can be created using Create->Quantum->Assets...
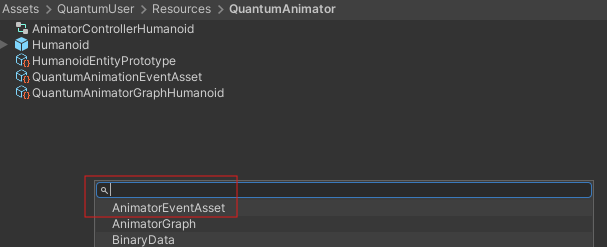
Instant Events will trigger only one time the Execute()
function once the Animator plays the clip that contains such event baked. A good pattern is to inherit from the base AnimatorInstantEventAsset
and override the base class methods with different procedures, like custom Frame.Siganls
.
C#
public unsafe class AnimatorInstantEventAsset : AnimatorEventAsset, IAnimatorEventAsset
{
public new AnimatorEvent OnBake(AnimationClip unityAnimationClip, AnimationEvent unityAnimationEvent)
{
var quantumAnimatorEvent = new AnimatorInstantEvent();
quantumAnimatorEvent.AssetRef = Guid;
quantumAnimatorEvent.Time = FP.FromFloat_UNSAFE(unityAnimationEvent.time);
return quantumAnimatorEvent;
}
public override void Execute(Frame f, AnimatorComponent* animator)
{
//Debug.Log("Execute Instant event.");
}
}
Event Setup
Follow these steps to define the event on an animation clip:
- Add the
AnimationEventData
component to the same GameObject the containsAnimator
component, in yourQuantumEntityPrototype
:

- On the Animation window select the clip and add a new
Unity AnimationEvent
:
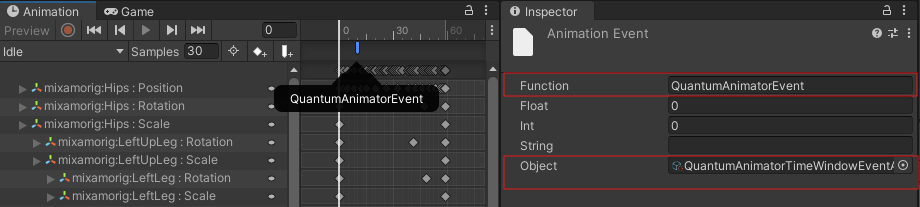
- Select the created event and specify the
Function
andObject
as the image below:
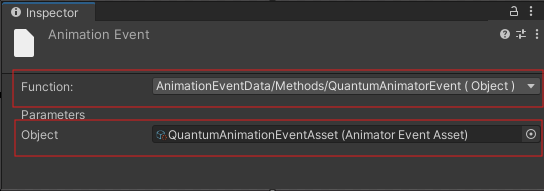
Creating a New Time-Window Event
The procedure is very similar to the instant event creation, the difference here is that you will need to create a second event of the same type in the Unity Clip to define the final of the event execution.
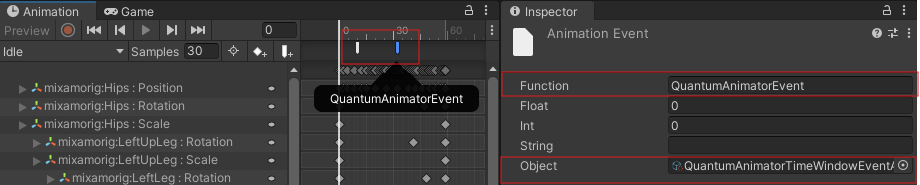
In case you want to create new events that have a similar behavior with OnEnter()
, Execute()
and OnExit()
methods, by inherit the AnimatorTimeWindowEventAsset
class:
C#
[Serializable]
public unsafe class AnimatorTimeWindowEventAsset : AnimatorEventAsset, IAnimatorEventAsset
{
public new AnimatorEvent OnBake(AnimationClip unityAnimationClip,
AnimationEvent unityAnimationEvent)
{
//...
}
public void OnEnter(Frame f, AnimatorComponent* animator)
{
//Debug.Log("OnEnter Time-window event.");
}
public override void Execute(Frame f, AnimatorComponent* animator)
{
//Debug.Log("Execute Time-window event.");
}
public void OnExit(Frame f, AnimatorComponent* animator)
{
//Debug.Log("OnExit Time-window event.");
}
}
Back to top